Ferdowsi University of Mashhad, Fall 2023 (1402 SH)
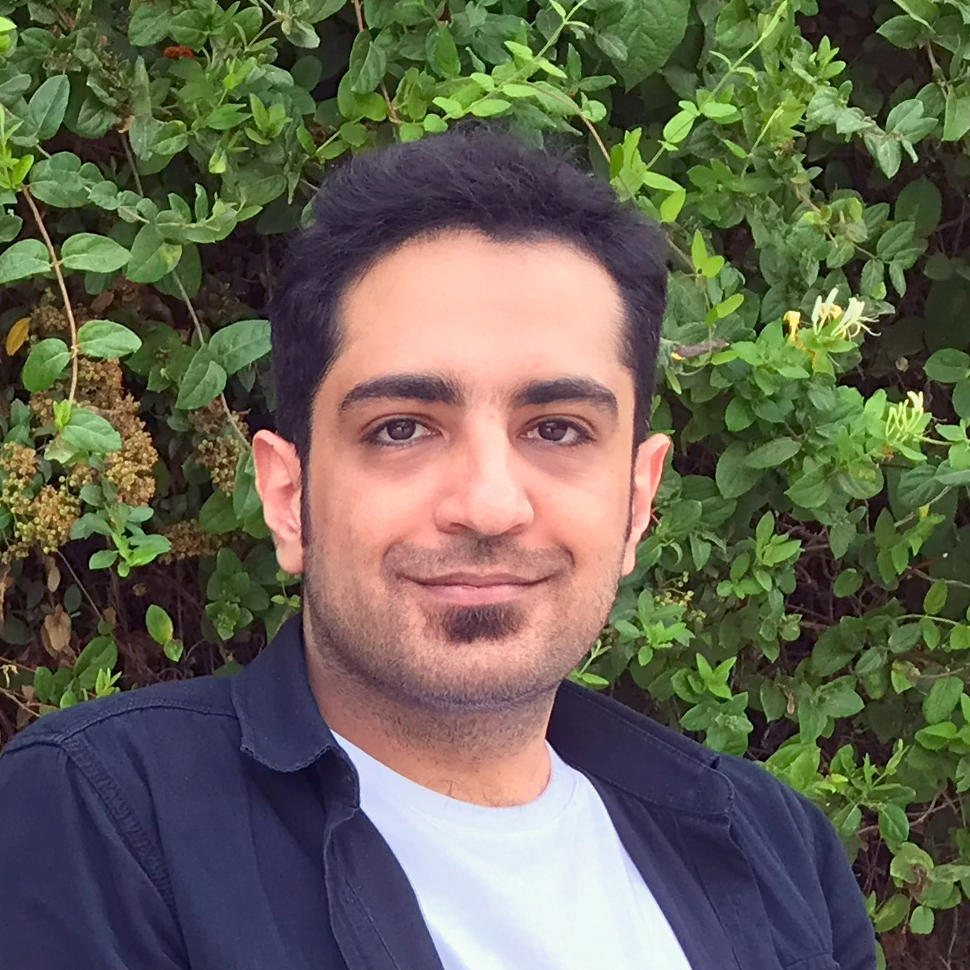
Welcome to the Course!
Lectures
Course Materials
- Presented PowerPoints - The majority of the course content is covered through class discussions and is comprehensively detailed on this webpage. However, for your convenience and further reference, the PowerPoint slides are also provided.
- Source Codes - Explore the source codes utilized throughout the course, available on GitHub.
- Final Project - Review the guidelines and details for the final project of the workshop.
Session 01 - Introduction and Course Overview
1402/08/02
Session 02 - Why Java and Hello World
1402/08/09
- Java’s Prominence - Over 1300 Languages Worldwide, ~700 Listed on Wikipedia
- Sun Microsystems’ Legacy: Java, Solaris OS, MySQL, SPARC, NFS, NetBeans, GlassFish, Sun Hardware
- Java Community Process (JCP) - And its big supporters: Apache Foundation (ASF), Eclipse, Google, Oracle, IBM, Red Hat, JetBrains
- Java Specification Requests (JSRs)
- Java Version History
- Java’s Platform Independence: Bytecode & JVM Mechanics
- How to Compile and Run Java Program
- Java Decompilation: Understanding Class Files & Main Methods
- JVM Optimizations: Function Inlining & Hotspot Compilation
Session 03 - Java Build Tools and RESTful Systems
1402/08/23
- Compiling Java Classes - Demonstrating with a “Hello World” example.
- Understanding Java Packages and Access Modifiers - Exploring default access and package relationships.
- Jar File Creation and Execution - Steps to package and run Java applications.
- Comparing Primitive and Reference Types - Analyzing differences and practical use cases.
- Introduction to Maven - Basics of Maven and library management via mvnrepository.com.
- JUnit Testing and Test-Driven Development (TDD) - Implementing palindrome function tests and exploring TDD principles.
- The TDD Cycle Explained - Emphasizing the importance of test-first development. See this GitHub repository for the class example.
- Basics of Remote Procedure Calls (RPC) - Understanding RPC and its TCP connections.
- The REST Architectural Style by Roy Fielding - An introduction to the concepts of REST.
- Key Specifications of RESTful Systems - Delving into statelessness, HTTP verbs (rfc7231), HTTP status codes, and cacheability.
- REST API Design: Best Practices and Examples - Simplifying system documentation with RESTful design. See examples from Box and Google Drive API.
- Further Reading on RESTful Best Practices: Microsoft’s Guidelines, Stack Overflow Insights, 13 Essential RESTful API Design Practices.
Session 04 - Building a College System using Spring Boot
1402/09/07
- Java SE vs Java EE - Understand the differences and applications.
- Spring Framework and Spring Boot - Introduction and project creation using start.spring.io and IntelliJ IDEA.
- Spring Boot Version Compatibility - Check Spring Boot and Java version compatibility.
- Creating a “Hello World” Endpoint in Spring Boot - Demonstrate a basic web application.
- Simplifying Code with Lombok - Enhance POJOs with Getters, Setters, and Constructors using Lombok annotations.
- Developing RESTful Services - Create a REST API for managing students.
- Assignment: College System - Implement a simple system with Student, Professor, and Person classes.
- Next session - Learn how to add Swagger, JPA, and H2 Database to the system.
Session 05 - Advancing the College Management System with Database Integration
1402/09/21
- System.out vs System.err - Explore the differences between standard output and error streams in Java.
- Understanding Log Levels - Discuss various log levels and the use of the SLF4J facade for flexible logging. SLF4J Manual
- Three-Layer Architecture - Describe the three-layer architecture and reorganize classes into three packages for clarity.
- Java Collections - Use Java Collections to store student data, transitioning from arrays to HashSet for improved performance.
- Builder Design Pattern - Understand the Builder design pattern and implement it using Lombok’s Builder annotation.
- Using H2 Database and its Console - Integrate an H2 database and its console for a simple database solution in Spring Boot 3.x.
- Transitioning to H2 Database and JPA - Replace Java data structures with H2 Database using JPA for enhanced data persistence. Also, you can view these videos to implement JPA: Video 1, Video 2, Video 3.
- The code we discussed in the class - Here is the code we discussed during the session.
Session 06 - Exploring Docker and Containerization in Development
1402/10/12
- What is Docker? - Introduction to Docker as an open-source platform for containerization.
- Differences between Docker and Virtual Machines - Understanding how Docker differs from traditional virtual machines.
- Benefits of Docker - Exploring the advantages of using Docker, such as consistent environments, application isolation, easy deployment, and resource efficiency.
- Installing Docker on Linux and Windows - Guide to installing Docker on various operating systems.
- Linux installation: Using Convenience Script
- Windows installation: Docker Desktop for Windows
- Understanding Docker Images - What constitutes a Docker image, including run commands, dependencies, and source code.
- Difference between Docker Image and Container - Clarifying the distinction between an image and a running container.
- Basic Docker Commands - Introduction to fundamental Docker commands like
docker images
,docker pull
,docker run
, anddocker ps
. - Creating a Dockerfile - We wrote a Dockerfile for the existing college-system example and built its image.
- Docker Compose Usage - Utilizing Docker Compose for running multi-container Docker applications.
- Optimizing Dockerfiles - Reduce Docker Image Size.
- Using Minimal Base Images - The importance of using minimalistic base images like Alpine for lighter Docker images. You can use
eclipse-temurin:17-jdk-alpine
for java. - Layer Caching in Docker - Understanding the caching mechanism in Docker and the importance of the order of layers in a Dockerfile.
- Reducing Docker Image Layers - Techniques to decrease the number of layers in a Docker image for optimization.
- Implementing Multi-Stage Builds - Using multi-stage builds in Docker to reduce image size and improve efficiency.
- Questions and Answers - Answers to questions that students asked during the session.
Session 07 - Aspect-Oriented Programming
1402/10/19
- Programming Paradigms in Different Languages - A comparison of programming paradigms in languages like JavaScript, Java, Python, C, and C++.
- Cross-Cutting Concerns in Software Development - Understanding cross-cutting concerns with a focus on Aspect-Oriented Programming.
- What Are Cross-Cutting Concerns? - Defining cross-cutting concerns in software development.
- Benefits of Aspect-Oriented Programming (AOP) - Exploring the advantages of using AOP, such as reuse, quick development, focus on one aspect, and easy enable/disable.
- AOP Concepts - Understanding the key concepts of AOP such as Pointcut, Advice, Aspect, Join Point, Weaver.
- Aspect-Oriented Programming example - Defining AOP and implementing it in Spring Boot. Read more here.
- Implementing a Time Logger with AOP - Using AOP to add a time logger to methods.
- Custom Annotation to use AOP - Discussing how annotations can be used to implement AOP in Spring Boot.
- Compile-Time vs. Runtime Weaving in AOP - Discussing compile-time weaving with AspectJ as an alternative to runtime weaving in Spring Boot AOP.
- Design Patterns in Software Development - Overview of design patterns like Decorator, Proxy, Singleton, and Facade.
- Polymorphism and Cyclomatic Complexity - How polymorphism can reduce cyclomatic complexity.
- The Diamond Problem in Java - Understanding the diamond problem and the introduction of interfaces in Java.